Scene rendering
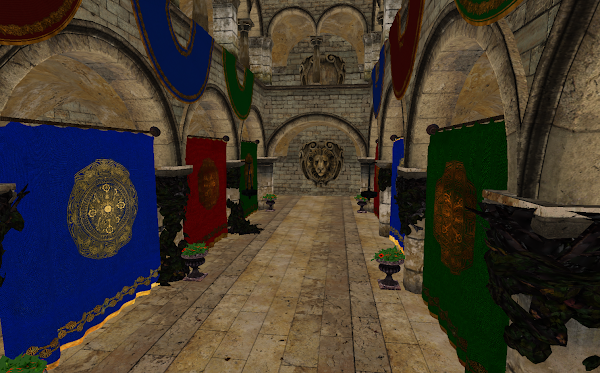
Now with the shaders compiled, vertex layout configured and descriptor set layouts specified, the rendering can begin. In a similar way as with the single triangle, you need to record draw calls. But this time a draw call per mesh will be recorded. Each draw call will automatically use the descriptor set of the individual material from the given mesh. But push constants as well as additional descriptor sets need to be configured in a callback if used (in the example here only the push constants get filled with individual data): auto recordMesh = [](const glm::mat4& mvp, // mvp matrix of mesh const glm::mat4& model, // model matrix of mesh vkcv::PushConstants& pushConstants, // push constants vkcv::Drawcall& drawcall // draw call ) { // append contents of the push constants for each draw call pushConstants.appendDrawcall(mvp); }; // record draw calls for the whole